Hello people..! So we finally come to the most famous part of Java, the Object Oriented Programming (OOP). Although I introduce OOP in this post, this post mainly to get you started with the notion of classes and objects. But, before we start our discussion on OOP, let us see why the modern world is so caught up with this programming paradigm. OOP offers you three important things –
- More Control – A program can be roughly divided into data (the variables) and code (the functions or the set of instructions). We know that the code performs some operations over the data. If we can somehow encapsulate the data and code such that certain data can be associated with only a certain code and no other code is allowed to touch the data directly, then we can easily find out which code is faulty. This gives us more control over the code… Wait… Not getting it..? 😛 … Let’s take up some sketches to make it clear…!
Code and Data in OOP Well, one obvious question is that why should you bother about all this..? You needn’t bother about having more control over your code if you are about to write 50 or 200 lines of code. But software which IT companies build, span up to millions of lines of code..! Yes, millions..! So, you need to have more control. And also because, the code is written by thousands of people. Imagine if you were to monitor 5 people. Would you use CCTV cameras..? No..! But if you were to monitor 1000 people..! Yes..! The same goes here.
- Re-usability – The Object Orientation feature Inheritance, allows us to reuse the existing code. This is a boon to companies building massive applications. If you can re-use the code properly, we can save a lot of time.
- Remember Less – The Object Orientation feature Polymorphism, enables us to remember less, thus making us more productive.
You will neither understand what they are, nor how they really work at this early stage. But we will learn them all very soon..! In this post, we will talk about the Java construct where all the OOP starts, the class.
The class
Class is a user defined data type. Think of it as a struct in C. Recall struct in C –
- It was a user defined data type.
- We could have any number of variables in a struct.
A class is also somewhat like that, only that it is much much more enhanced. Before we go ahead and learn the extra things, let us look at the syntax of a class with the features we are already familiar with.
// Declare using // 'class' keyword class Person { // These are variables or // attributes of the class String name; String phone; String email; int age; }
That is the syntax for creating a class. We generally don’t put an ending semicolon after the class declaration (like as we do with struct in C ), but it is ok if you do.
Now, we can’t have functions in a C struct. But we can have functions (methods) in a class. If so, when are they called? We’ll look at it later. Right now, let’s just focus on the syntax for writing methods in a class –
// Declare using // 'class' keyword class Person { // Instance Variables String name; String phone; String email; int age; // The usual syntax - // return-type function-name(parameters) { // code } void printDetials() { // Instance Variables are // accessible to every method System.out.println("Name - " + name); System.out.println("Phone - " + phone); System.out.println("Email - " + email); System.out.println("Age - " + age); } }
Using that syntax, you can write any number of methods in a class. Now, I have used a new term, Instance Variables. We will discuss later what this actually means. Just know that we can access them from the methods.
Entity
Now we can’t create a class as we like. A class in your code generally represent an entity in the real world (not necessarily living). We created a class person, and we know that a person exists in the real world. Like that, your class must represent something in the real world, or it must represent an entity. So, what do we do in a class…? In a class, we describe what the entity must do..! Every entity has two things –
- Attributes (Instance Variables) – Just like every Person has a name and age, every entity is supposed to have some attributes or properties.
- Behavior (Methods) – Every Person would perform some action, say, walk, sneeze, laugh, etc., in code, they are written as methods.
We must specify these. Remember, we are still specifying..! That is, we are still describing what your entity does. Which kinda means that we are still designing the entity, we haven’t yet created an instance of it.
Examples
Let us take up some real life entities and try to represent them as classes so that we can understand, what we should write as attributes and methods. Let us first take a very simple example, a ball.
class Ball { // Attributes int innerRadius, outerRadius, weight; // Actions void bounce() { // some code } }
There isn’t much in a ball, so this is fine. Now, let’s look at something a little more complex.
class Animal { // Attributes int height, weight, color; // Actions void eat() { System.out.println("Animal is eating..."); } void sleep() { System.out.println("Animal is sleeping..."); } void walk() { System.out.println("Animal is walking..."); } }
Now that’s a small animal of the wild..! How about something even more complex?
class SavingsAccount { // Attributes String accountNumber; int balance; double interestRate = 10.5; // Actions void withdraw(int fund) { if (balance - fund >= 0) { balance -= fund; } } void deposit(int fund) { if (fund > 0) { balance += fund; } } void addInterest() { balance += (balance * interestRate) / 100; } }
All these were just to give you a hint on how we write classes. These entities can have a lot more attributes and actions. I have mentioned only a few so that I can keep it simple. Try adding more attributes and actions..! 😉
Objects
So far, what we have seen is a class. Class is as we discussed, is a data type. We don’t store values or data in a data type, we store data in objects! An object is a live instance or occurrence of a class. Memory is allocated when we create an object. We create an object using the new keyword. When we create an object using the new keyword, a chunk of memory is allocated and a reference to that chunk of memory is returned.
How big is this chunk of memory. Well, let’s just say that it is big enough to accommodate all the attributes of the class. Before we go to the syntax of creating an object, let’s look at a couple of definitions.
Object Reference – It is a variable which holds the address or reference to the object. We access the elements of an object via the Object Reference. We use the de-referencing, or dot operator ‘.’ to access the members of the object. An Object Reference has a data type. It has the same data type as the class whose object we want the object reference to point to.
Object – It is one large chunk of memory dynamically allocated by using the new keyword. It is the live instance of a class. It has memory for variables in it. They are the variables which are specified in the class definition. Object is a reference type. So they must be accessed by a pointer sort of thing, which in Java is an Object Reference.
Now, these definitions will make more sense once we go through some examples. This is how we create an object of Ball class –
// Creating an object reference of type Ball Ball oref; // Creating an object and assigning // the reference of the object oref = new Ball();
Remember, Ball was a class. So it is a data type (user-defined). Visually, it looks something like –
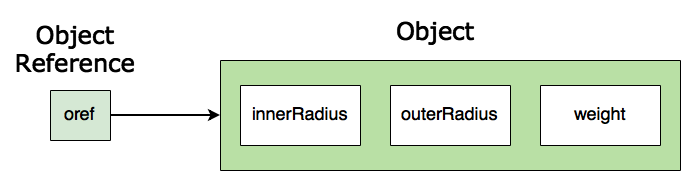
So, the object reference points to the object. In the diagram, the object is having only 3 variables, that is because we had 3 variables in the class definition. If you change that, the object also changes. Class is the blueprint and Object is the live occurrence. Any changes made to the blueprint is reflected in the model.
We can access the members of the object via the object reference –
// We access the attributes by the object reference System.out.println(oref.innerRadius); System.out.println(oref.outerRadius); System.out.println(oref.weight); // We also access the Actions / Methods // by the object reference oref.bounce();
So, if we put all the pieces together –
class Ball { // Attributes int innerRadius, outerRadius, weight; // Actions void bounce() { System.out.println("Bounce..!"); } } class TestBall { public static void main(String[] args) { // Creating an object reference of type Ball Ball oref; // Creating an object and assigning // the reference of the object oref = new Ball(); // We access the attributes by the object reference System.out.println(oref.innerRadius); System.out.println(oref.outerRadius); System.out.println(oref.weight); // We also access the Actions / Methods // by the object reference oref.bounce(); } }
The output of that piece of code is –
0 0 0 Bounce..!
So, this was to get you started with classes and objects. We will learn a lot more about classes and objects as we keep learning more and more of Java. Programmers who never dealt with OOP before will find this hard to digest. But trust me, it is worth the pain..! Keep practising… Happy Coding..! 😀
One thought on “Java Tutorials – Classes and Objects”
Hi Vamsi,
Really awesome way of explanation.
Just a doubt, Here we are calling a method (oref.bounce();) from main (that is static) but why we didnt give static in the function declaration (void bounce ()).
It should be like static void bounce() right ?
Just I recollected from ” if you want to call any method from the main() method, it must be marked static, otherwise it is a compilation error..!”
Thanks,
Anilkumar Nagamani